FilePresentationService
The FilePresentationService allows presenting files during a conference. The Dolby.io Communications APIs service converts the user-provided file into multiple pages, as images, accessible through the image method.
The file presentation workflow:
1. The presenter calls the convert method to upload and convert a file.
2. The presenter receives the conversionProgress event that informs that the file conversion is in progress.
3. The presenter receives converted event when the file conversion is finished.
4. The presenter calls the start method to start presenting the file.
5. The presenter and the viewers receive the started event that informs that the file presentation starts. Upon receiving the started event, call the image method to get the converted file images URLs and display the proper page of the file by retrieving the individual images.
6. The application is responsible for coordinating the page flip between the local and the presented files. The presenter calls the update method to inform the service to send the updated page number to the participants.
7. The presenter and viewers receive the updated event with the current page number. Receiving the updated event should trigger calling the image method to display the proper page of the file by retrieving the individual images.
8. The presenter may call the thumbnail method to obtain thumbnail images of the file and implement a carousel control for the presenting user to flip pages locally.
9. The presenter calls the stop method to end the file presentation.
10. The presenter and the viewers receive the stopped event to inform about the end of the file presentation.
The following diagram presents the easiest workflow for presenting files during a conference.
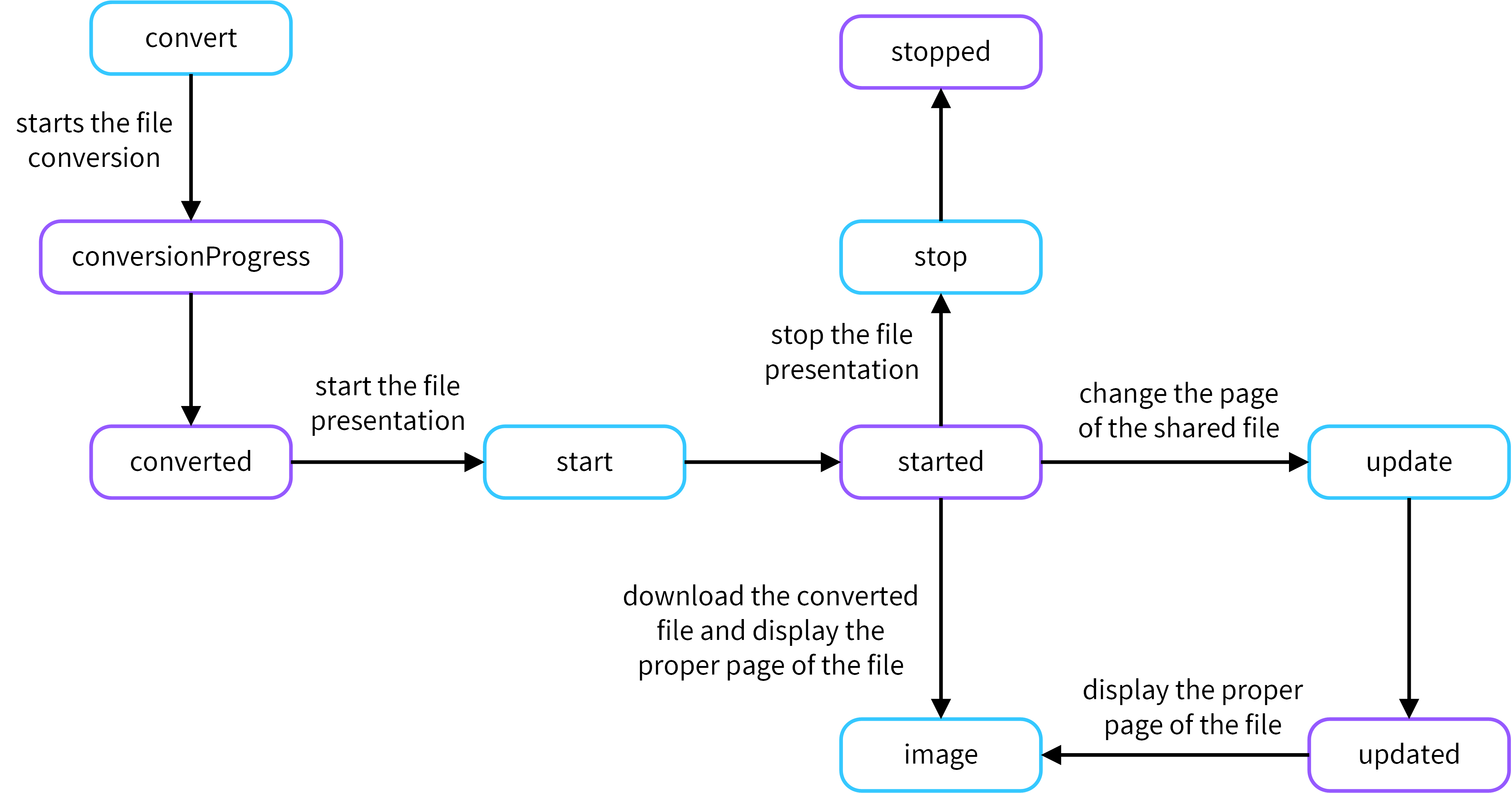
The file presentation diagram
The current accessor allows the participants to receive information about the current state of the file presentation.
Events
started
• started(e
: FilePresentation): void
Emitted when the presenter started the file presentation.
example
VoxeetSDK.filePresentation.on("started", (e) => {});
Parameters:
Name | Type | Description |
---|---|---|
e | FilePresentation | The object containing properties specific to the event. |
Returns: void
stopped
• stopped(e
: FilePresentation): void
Emitted when the presenter ended the file presentation.
example
VoxeetSDK.filePresentation.on("stopped", (e) => {});
Parameters:
Name | Type | Description |
---|---|---|
e | FilePresentation | The object containing properties specific to the event. |
Returns: void
conversionProgress
• conversionProgress(e
: FileConversionProgress): void
Emitted when the presenter started converting the file and the file conversion is in progress.
example
VoxeetSDK.filePresentation.on("conversionProgress", (e) => {});
Parameters:
Name | Type | Description |
---|---|---|
e | FileConversionProgress | The object containing properties specific to the event. |
Returns: void
converted
• converted(e
: FileConverted): void
Emitted when the file is converted.
example
VoxeetSDK.filePresentation.on("converted", (e) => {});
Parameters:
Name | Type | Description |
---|---|---|
e | FileConverted | The object containing properties specific to the event. |
Returns: void
updated
• updated(e
: FilePresentation): void
Emitted when the presenter changed the displayed page of the shared file. The event includes information about the current page number.
example
VoxeetSDK.filePresentation.on("updated", (e) => {});
Parameters:
Name | Type | Description |
---|---|---|
e | FilePresentation | The object containing properties specific to the event. |
Returns: void
Accessors
current
• get current(): FilePresentation
Returns information about the current state of the file presentation. Use this accessor if you wish to receive information that is available in the FilePresentation object, such as the file ID, the number of images in the presentation, information about the file owner, or the current position in the presentation. For example, use the following code to ask about the file owner:
VoxeetSDK.filePresentation.current.owner;
Returns: FilePresentation
Methods
convert
▸ convert(file
: File): Promise<XMLHttpRequest>
Converts the user-provided file into multiple pages, as images, that can be shared during the file presentation. The file is uploaded as FormData. The maximum possible size of the uploaded file is 250 MB. In the case of uploading a file that exceeds 250 MB, the SDK triggers an error.
Supported file formats are:
- doc/docx (Microsoft Word)
- ppt/pptx
After conversion, the files are broken into individual images with maximum resolution capped at 2560x1600.
When the file is converted and ready for the presentation, the application receives the converted event.
Only the converted files can be shared during conferences.
Parameters:
Name | Type | Description |
---|---|---|
file | File | The file that the presenter wants to share during the conference. |
Returns: Promise<XMLHttpRequest>
image
▸ image(page
: number, fileId?
: string): Promise<string>
Provides the image's URL that refers to a specific page of the presented file.
Parameters:
Name | Type | Description |
---|---|---|
page | number | The number of the presented page. Files that do not have any pages, for example jpg images, require setting the value of the page parameter to 0 . |
fileId? | string | The file ID that allows presenting files without joining a conference. |
Returns: Promise<string>
start
▸ start(file
: FileConverted): Promise<any>
Starts a file presentation. The Dolby.io Communications APIs allow presenting only the converted files.
This method is not available for listeners. Calling this method by a listener results in the UnsupportedError.
Parameters:
Name | Type | Description |
---|---|---|
file | FileConverted | The converted file that the presenter wants to share during the conference. |
Returns: Promise<any>
stop
▸ stop(): Promise<any>
Stops the file presentation.
Returns: Promise<any>
thumbnail
▸ thumbnail(page
: number, fileId?
: string): Promise<string>
Provides the thumbnail's URL that refers to a specific page of the presented file.
Parameters:
Name | Type | Description |
---|---|---|
page | number | The number of the presented page. Files that do not include any pages, for example jpg images, require setting the value of this parameter to 0 . |
fileId? | string | The file ID that allows presenting files without joining a conference. |
Returns: Promise<string>
update
▸ update(page
: number): Promise<any>
Informs the service to send the updated page number to the conference participants.
Parameters:
Name | Type | Description |
---|---|---|
page | number | The page number that corresponds to the page that should be presented. |
Returns: Promise<any>
Updated about 1 year ago