FilePresentationService
The FilePresentationService allows presenting files during a conference. The Dolby.io Communications APIs service converts the user-provided file into multiple pages, as images, accessible through the image method.
The file presentation workflow:
1. The presenter calls the convert method to upload and convert a file.
2. The presenter calls the start method to start presenting the file.
3. The presenter and the viewers receive the FilePresentationStarted event that informs that the file presentation starts. Upon receiving the FilePresentationStarted event, call the image method to get the converted file images URLs and display the proper page of the file by retrieving the individual images.
4. The application is responsible for coordinating the page flip between the local and the presented files. The presenter calls the update method to inform the service to send the updated page number to the participants.
5. The presenter and viewers receive the FilePresentationUpdated event with the current page number. Receiving the FilePresentationUpdated event should trigger calling the image method to display the proper page of the file by retrieving the individual images.
6. The presenter may call the thumbnail method to obtain thumbnail images of the file and implement a carousel control for the presenting user to flip pages locally.
7. The presenter calls the stop method to end the file presentation.
8. The presenter and the viewers receive the FilePresentationStopped event to inform about the end of the file presentation.
The following diagram presents the easiest workflow for presenting files during a conference.
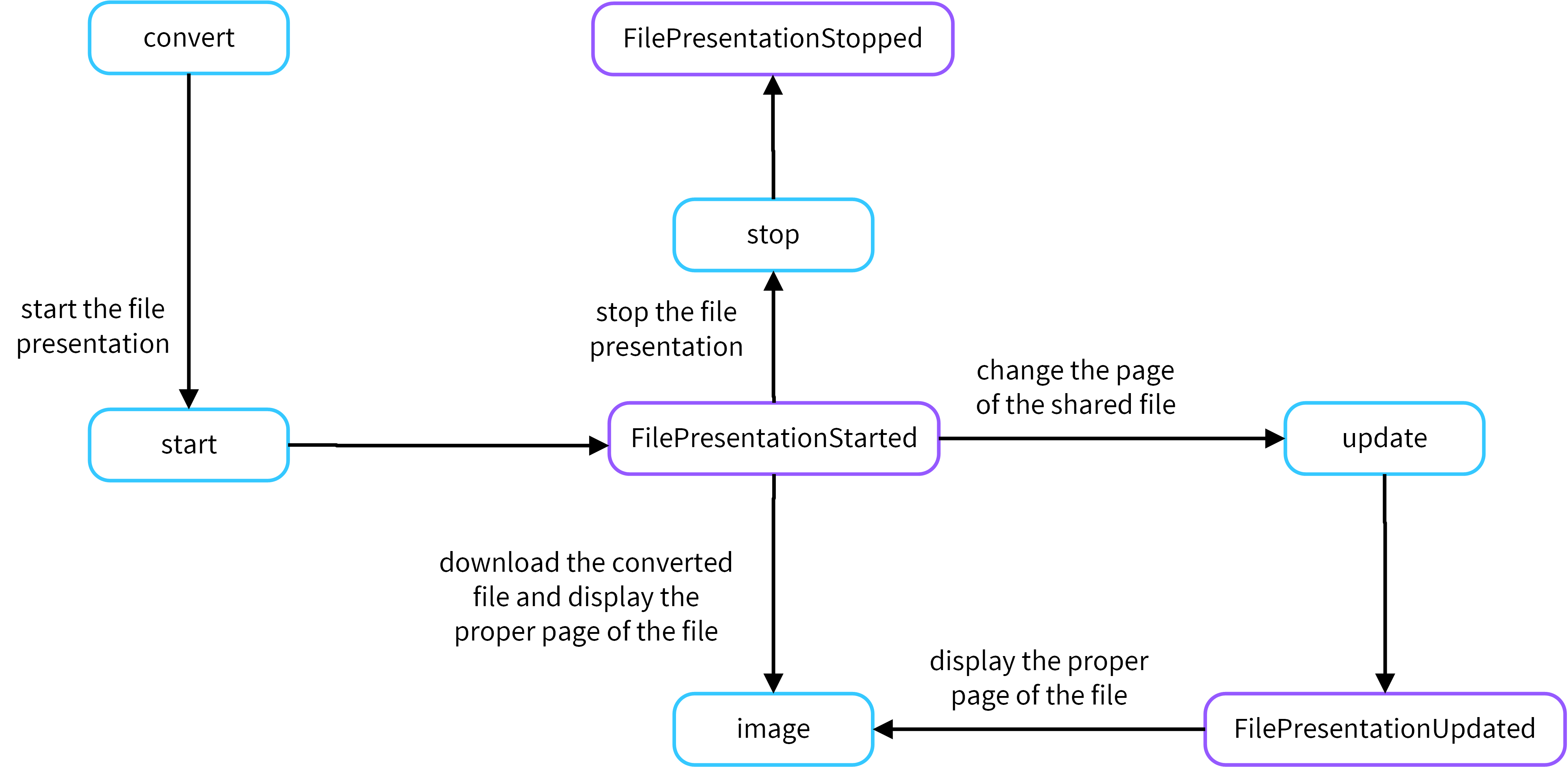
The file presentation diagram
The service is available only to participants who joined a conference using the join method; it is not available for listeners.
Available in the package com.voxeet.sdk.services.FilePresentationService.
Methods
image
▸ image(fileId
: String, pageNumber
: int): String
Provides the image's URL that refers to a specific page of the presented file.
Parameters
Name | Type | Description |
---|---|---|
fileId | String | The ID of the presented file. |
pageNumber | int | The page number that corresponds to the currently presented page of the shared file. |
Returns: @Nullable String - the formatted string.
thumbnail
▸ thumbnail(fileId
: String, pageNumber
: int): String
Provides the thumbnail's URL that refers to a specific page of the presented file.
Parameters
Name | Type | Description |
---|---|---|
fileId | String | The ID of the presented file. |
pageNumber | int | The page number that corresponds to the currently presented page of the shared file. |
Returns: @Nullable String - the formatted string.
convert
▸ convert(@NonNull file
: File): Promise<FilePresentation>
Converts the user-provided file into multiple pages, as images, that can be shared during the file presentation. The file is uploaded as FormData.
Supported file formats are:
- doc/docx (Microsoft Word)
- ppt/pptx
After conversion, the files are broken into individual images with maximum resolution capped at 2560x1600.
Only the converted files can be shared during conferences.
Parameters
Name | Type | Description |
---|---|---|
file | File | non null valid The file that the presenter wants to share during the conference. |
Returns: @NonNull Promise<FilePresentation> - the promise to resolve.
start
▸ start(@NonNull body
: FilePresentationConverted): Promise<FilePresentation>
Starts a file presentation. The Dolby.io Communications APIs allow presenting only the converted files.
Parameters
Name | Type | Description |
---|---|---|
body | FilePresentationConverted | non null The valid descriptor of the file. |
Returns: @NonNull Promise<FilePresentation> - the promise to resolve.
start
▸ start(@NonNull body
: FilePresentationConverted, position
: int): Promise<FilePresentation>
Starts a file presentation. The Dolby.io Communications APIs allow presenting only the converted files. This method allows presenting a specific page of the converted file.
Parameters
Name | Type | Description |
---|---|---|
body | FilePresentationConverted | non null The valid descriptor of the file. |
position | int | The page number that should be presented. |
Returns: @NonNull Promise<FilePresentation> - the promise to resolve.
stop
▸ stop(@NonNull fileId
: String): Promise<FilePresentation>
Stops the file presentation.
Parameters
Name | Type | Description |
---|---|---|
fileId | String | non null The ID of the presented file. |
Returns: @NonNull Promise<FilePresentation> - the promise to resolve.
update
▸ update(@NonNull fileId
: String, position
: int): Promise<FilePresentation>
Informs the service to send the updated page number to the conference participants.
Parameters
Name | Type | Description |
---|---|---|
fileId | String | non null The ID of the presented file. |
position | int | The page number that corresponds to the page that should be presented. |
Returns: @NonNull Promise<FilePresentation> - the promise to resolve.
Events
FilePresentationStarted
Emitted when the presenter started the file presentation.
This object can be accessed through the WebSocket usage.
Available in the package com.voxeet.sdk.json.FilePresentationStarted.
conferenceId (String)
The conference ID.
userId (String)
The file owner's ID.
imageCount (int)
The number of images of the presented file.
fileId (String)
The file ID.
position (int)
The currently displayed page of the shared file.
FilePresentationStopped
Emitted when the presenter ended the file presentation.
This object can be accessed through the WebSocket usage.
Available in the package com.voxeet.sdk.json.FilePresentationStopped.
conferenceId (String)
The conference ID.
userId (String)
The file owner's ID.
fileId (String)
The file ID.
FilePresentationUpdated
Emitted when the presenter changed the displayed page of the shared file. The event includes information about the current page number.
This object can be accessed through the WebSocket usage.
Available in the package com.voxeet.sdk.json.FilePresentationUpdated.
conferenceId (String)
The conference ID.
userId (String)
The file owner's ID.
fileId (String)
The file ID.
position (int)
The currently displayed page of the shared file.
Updated 8 months ago